Retry mechanisms, event scheduling or session expiration. All situations where you might want to create new time value some “duration” after an earlier time value.
This is where the Add
method on the time.Time
type comes in.
It creates a new time.Time
some specific duration after the original time.Time
value. If plotted on a timeline this essentially is essentially a “move forward”.
t1 + 2 seconds = t2
In Go, a time.Duration
value has a resolution of 1 nanosecond. You can multiple it with the available “unit” constants to convert to seconds, hours and days. See the examples below.
Adding seconds
In the example below we create t2
by adding 2 seconds to t1
.
package main
import (
"fmt"
"time"
)
func main() {
t1 := time.Date(2024, 2, 8, 13, 37, 11, 0, time.UTC)
t2 := t1.Add(2 * time.Second)
fmt.Println(t1.String())
fmt.Println(t2.String())
}
Adding hours
This works similarly for hours, if we want t2
to be 3 hours after t1
, we can do the following.
package main
import (
"fmt"
"time"
)
func main() {
t1 := time.Date(2024, 2, 8, 13, 37, 11, 0, time.UTC)
t2 := t1.Add(3 * time.Hour)
fmt.Println(t1.String())
fmt.Println(t2.String())
}
Adding days
You won’t find a time.Day
constant in the time
package. Mainly because this is where “ambiguity sets in”. Due to timezones and daylight saving time, days will not always take exactly 24 hours. In such situations you might want to look at t.AddDate
.
However, if you are working in UTC (where every day does have 24 hours), you can use the following code.
package main
import (
"fmt"
"time"
)
func main() {
t1 := time.Date(2024, 2, 8, 13, 37, 11, 0, time.UTC)
t2 := t1.Add(24*time.Hour)
fmt.Println(t1.String())
fmt.Println(t2.String())
}
Please note that this is still not entirely correct due to leap seconds. But the Go time
package does not support those to start with, so I’m skipping them here as well.
Negative duration
By providing a negative duration, you can subtract durations from time.Time
values.
Location
The time.Time
values created by the Add
method will always be in the same location as the original time value.
Monotonic clock
If the original time.Time
value is created via time.Now()
, it will often contain a monotonic element. Calling Add
on such
a value will create a new value that also contain a monotonic element. With the monotonic element adjusted for the duration provided to Add
.
This is the only way to create new time.Time
values that have monotonic elements without calling time.Now()
.
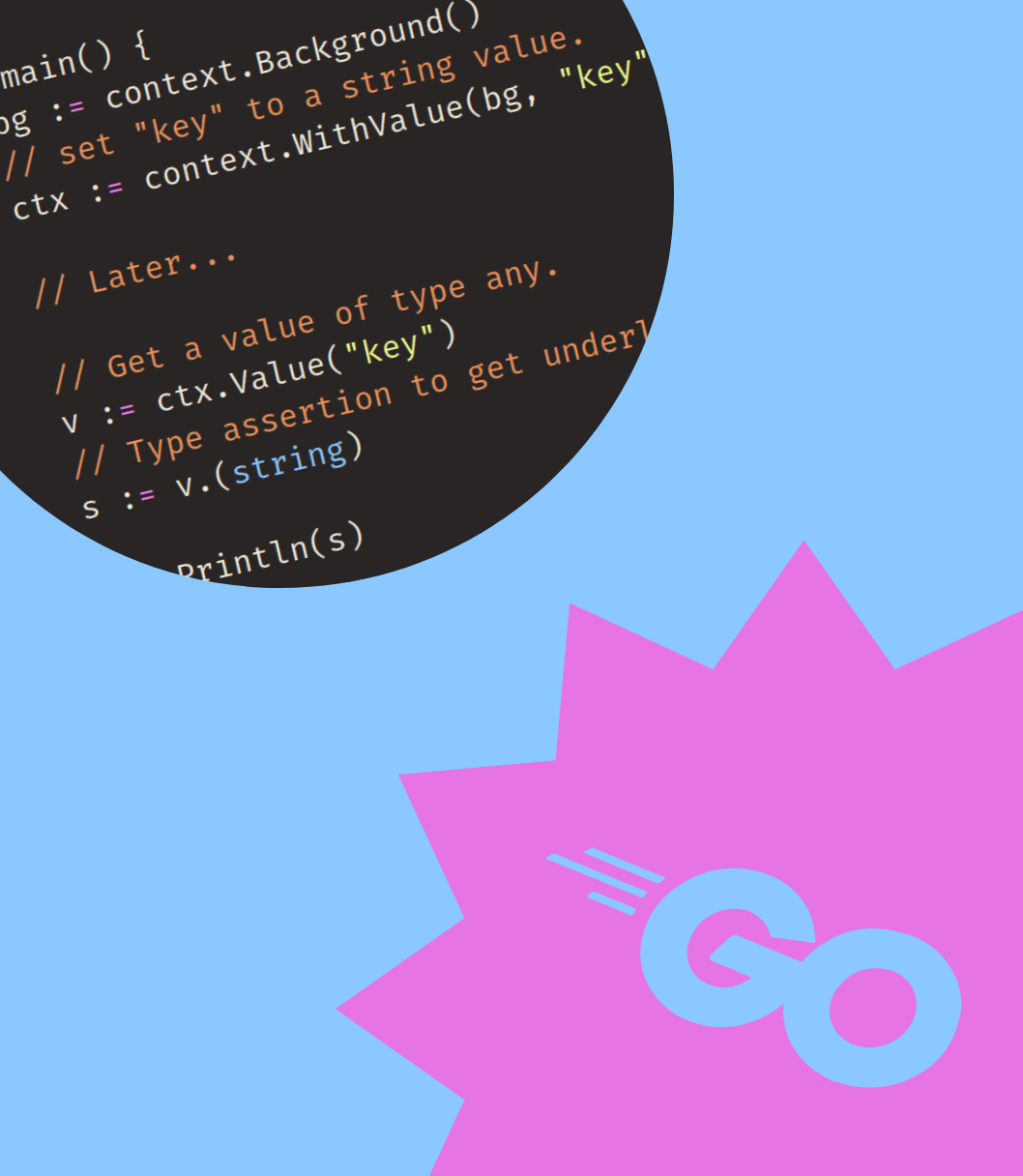
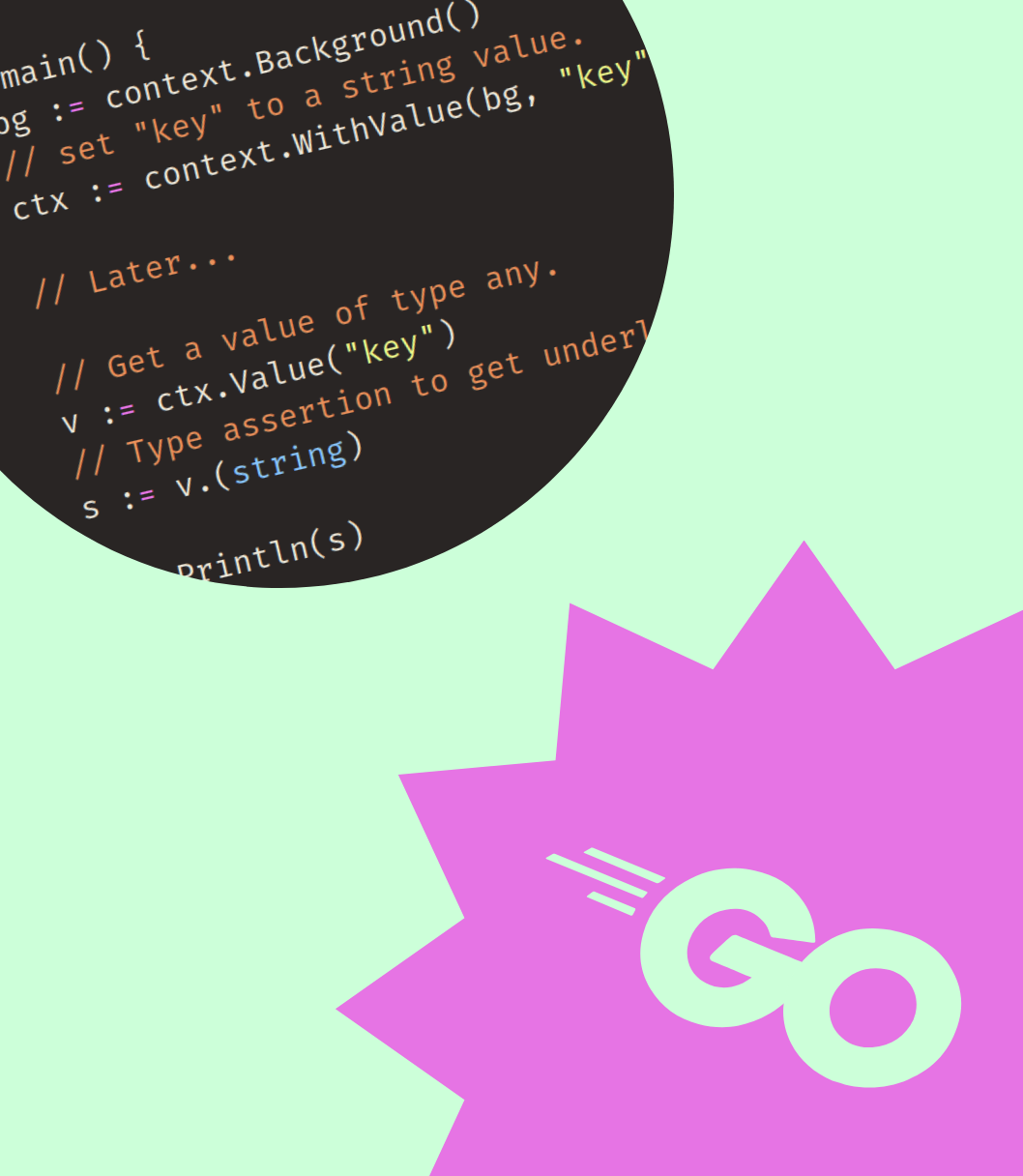
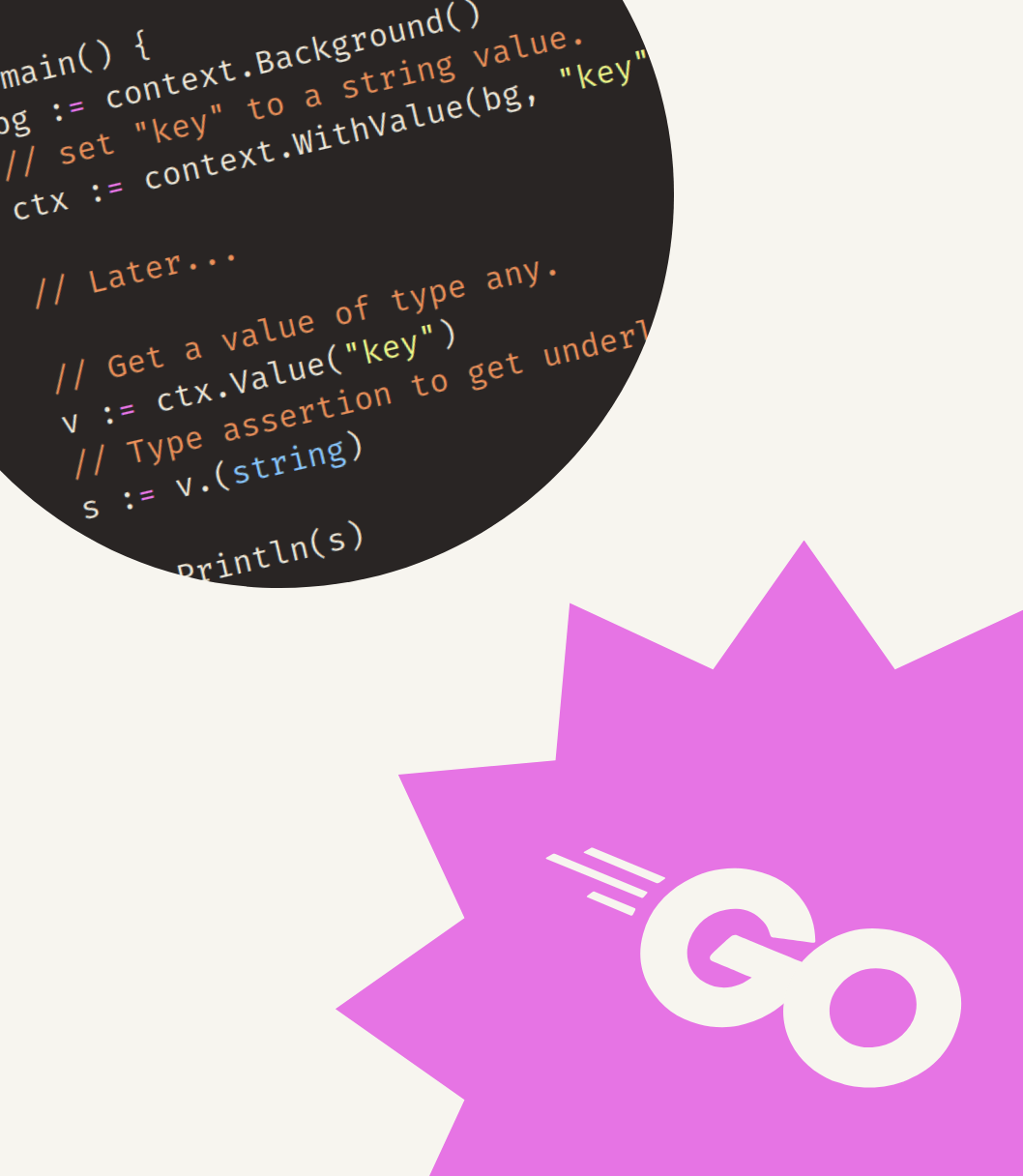
Get my free newsletter periodically*
Used by 500+ developers to boost their Go skills.
*working on a big project as of 2025, will get back to posting on the regular schedule once time allows.
