Directly deleting an element from a slice is not possible in Go. However, you can overwrite the element with later elements by using append
.
s = append(s[:i], s[i+1:]...)
This should be read as: append “all elements that come after i
” to “the elements up to i
”.
Due to the way append works, this will not allocate a new backing array.
append
, otherwise your s
slice will contain duplicate data. See the last two elements in the backing array in the example below.
As a more concrete example, suppose you have the following food
slice backed by array a
.
a := [6]string{"", "🍎", "🍔", "🌭", "🍕", ""}
food := a[1:5]
To delete the element with index 1 (index 2 in the backing array) we would write the following code:
food = append(food[:1], food[2:])
If we diagram the steps taken, it would look like this:
Depending on your Go version there might already be a function that implements this code:
- In 1.21 and later, use the
Delete
function in theslices
package in the standard library. - For earlier versions, you can find the same function in the experimental
slices
package (also shown in the example below).
If you are using a Go version that does not support generics, you can always implement your own Delete
function. See the example below:
package main
import (
"fmt"
"slices"
)
func main() {
x := []string{"👁", "👃", "👁"}
y := []string{"👁", "👃", "👁"}
fmt.Println("x and y before", x, y)
x = Delete(x, 1)
y = slices.Delete(y, 1, 2)
fmt.Println("x and y after", x, y)
}
func Delete(s []string, i int) []string {
return append(s[:i], s[i+1:]...)
}
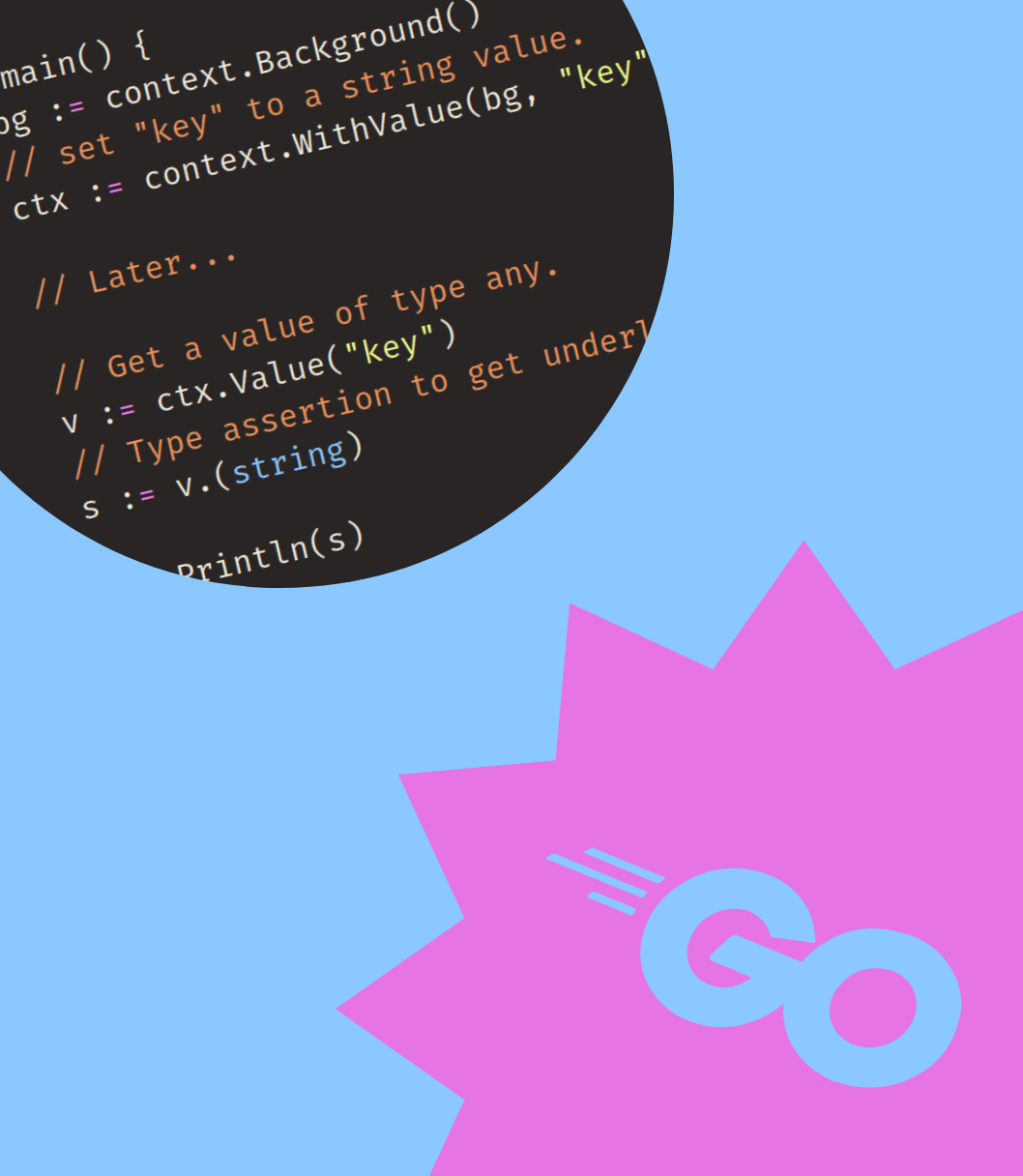
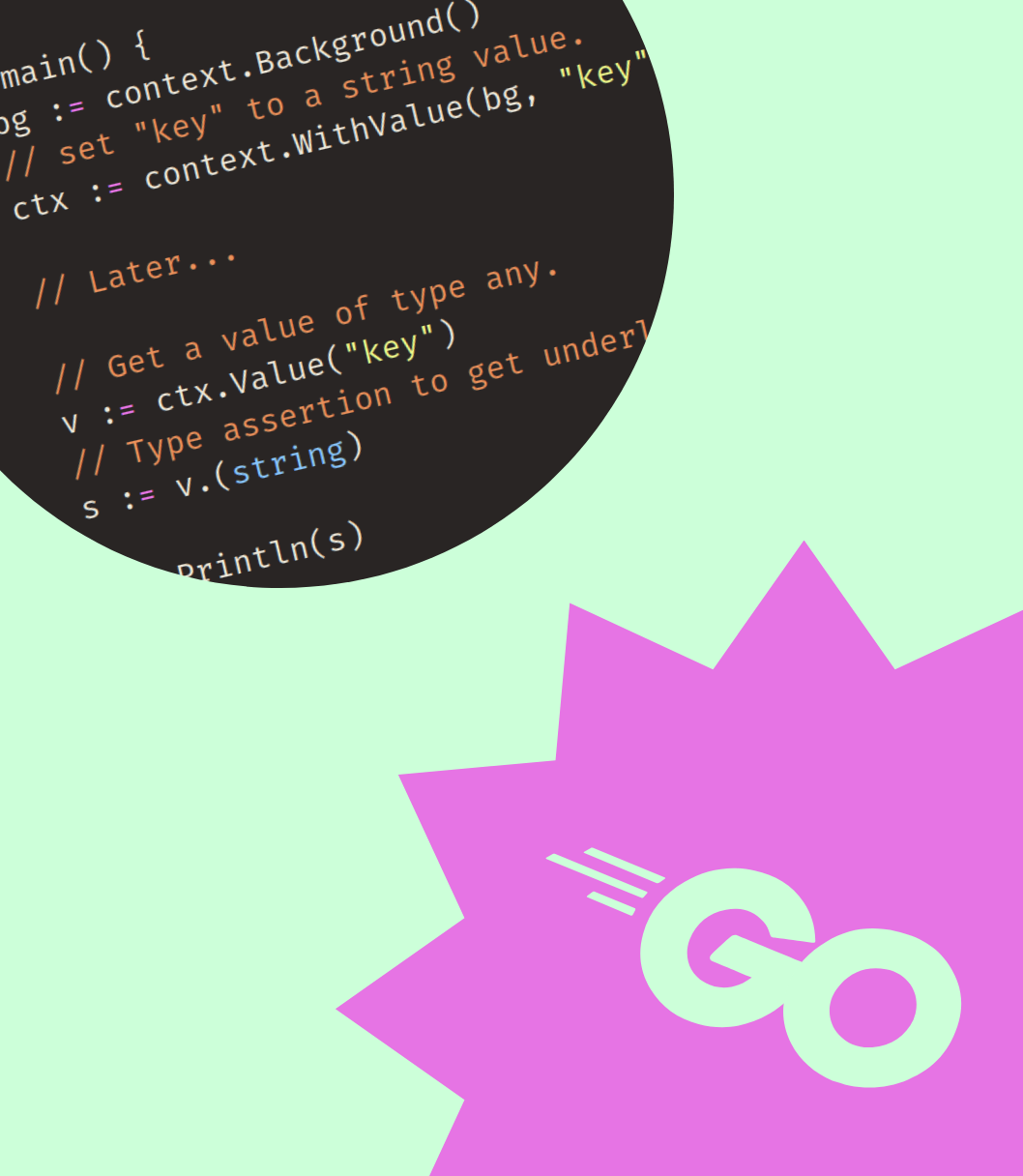
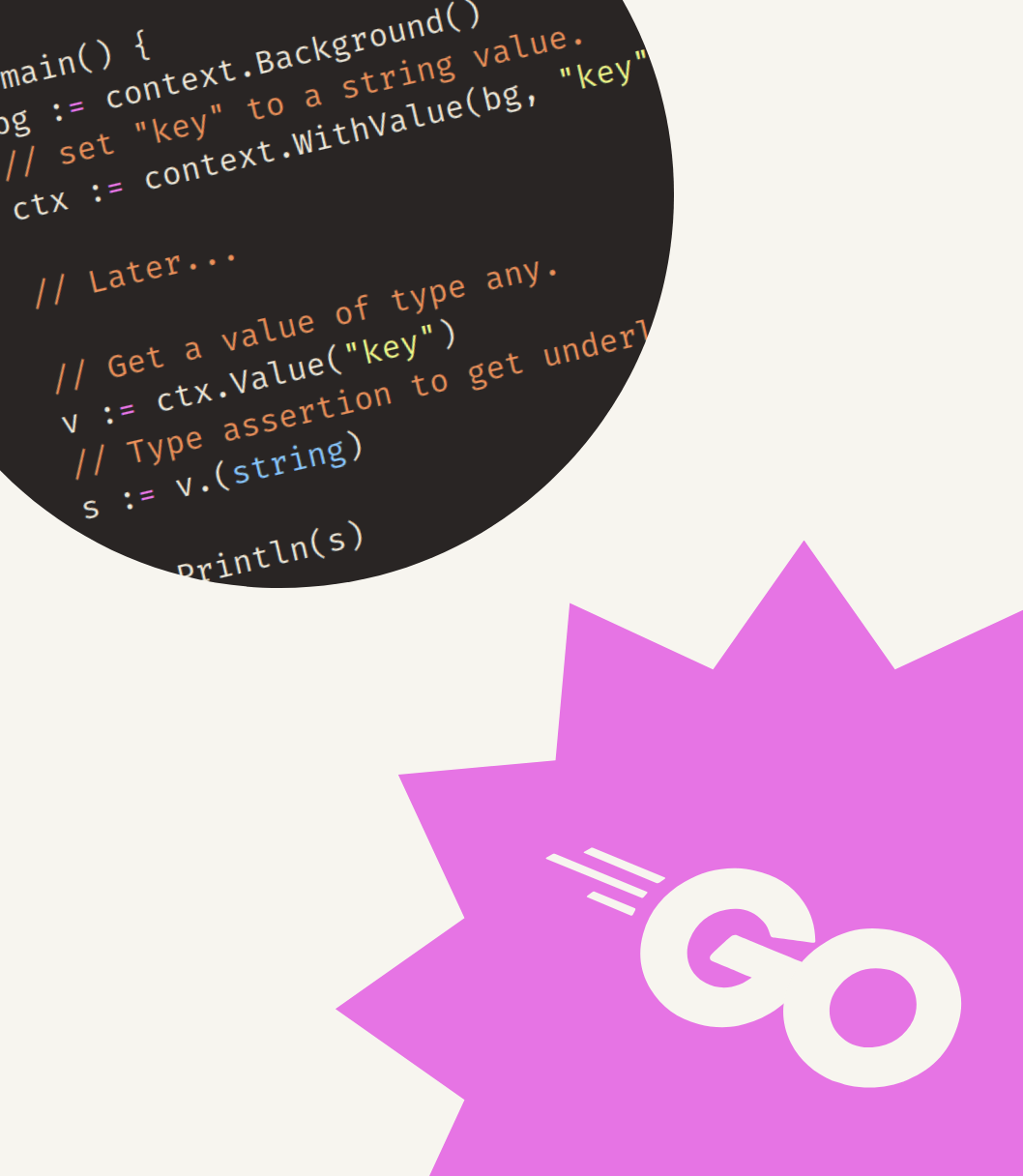
Get my free newsletter every second week
Used by 500+ developers to boost their Go skills.
"I'll share tips, interesting links and new content. You'll also get a brief guide to time for developers for free."
