If for any reason you explicitly need a consistent offset from UTC you probably want to use “fixed zone” Locations.
“Fixed” in this case means that the resulting timezone will not be influenced by Daylight Saving Time (DST) or other changes.
This snippet will show you how to create a fixed timezone using the time.FixedZone
function and then use it to represent a time instant.
Before we dive in, it’s good to know how time.Time
values represent time instants. Learn more here.
Change to a specific UTC offset
The time.FixedZone
function creates a new time.Location
reference with a fixed offset from UTC.
By passing this reference to the t.In(loc *time.Location)
method we can create a new time.Time
value in that location.
For example:
package main
import (
"fmt"
"time"
)
func main() {
// t1 represents a time instant in UTC.
t1 := time.Date(2024, 01, 22, 13, 37, 0, 0, time.UTC)
// create a timezone 2 hours west of UTC
westOfUTC := time.FixedZone("UTC-2", -2*60*60)
// t2 represents the same time instant in UTC-2
t2 := t1.In(westOfUTC)
fmt.Println(t1.String())
fmt.Println(t2.String())
fmt.Printf("same time instant: %v\n", t1.Equal(t2))
}
Here we initially have a t1
value in the UTC
timezone.
We then use time.FixedZone
to create a new location called UTC-2
. This location is always 2 hours west of UTC
.
Finally, we use t1.In(westOfUTC)
to create t2
. This time.Time
value will be in the UTC-2
timezone.
t1
and t2
represent the same time instant, but they will have different clock values. t2
will read 2 hours before t1
.
If you run the example you can see that’s the case.
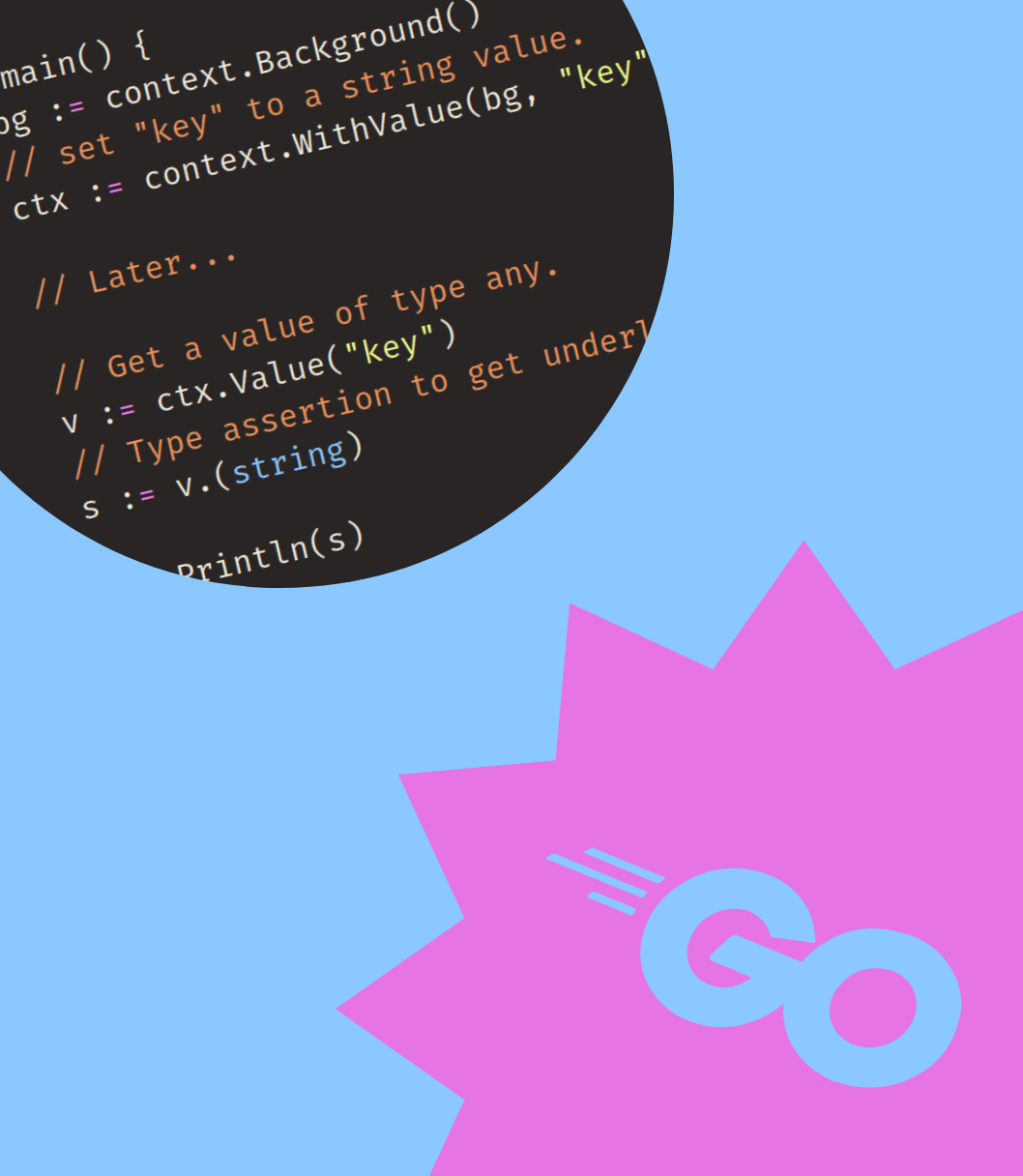
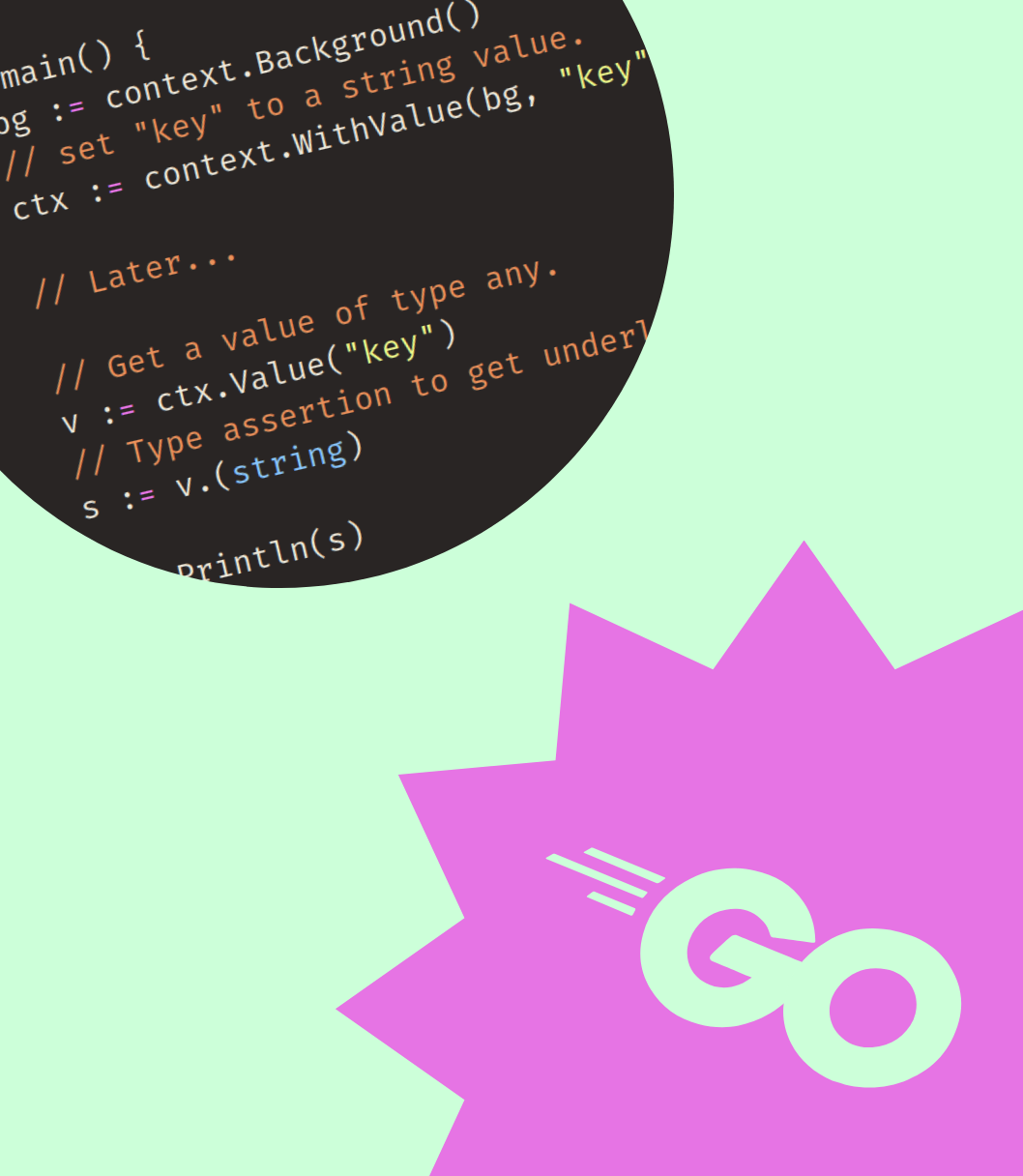
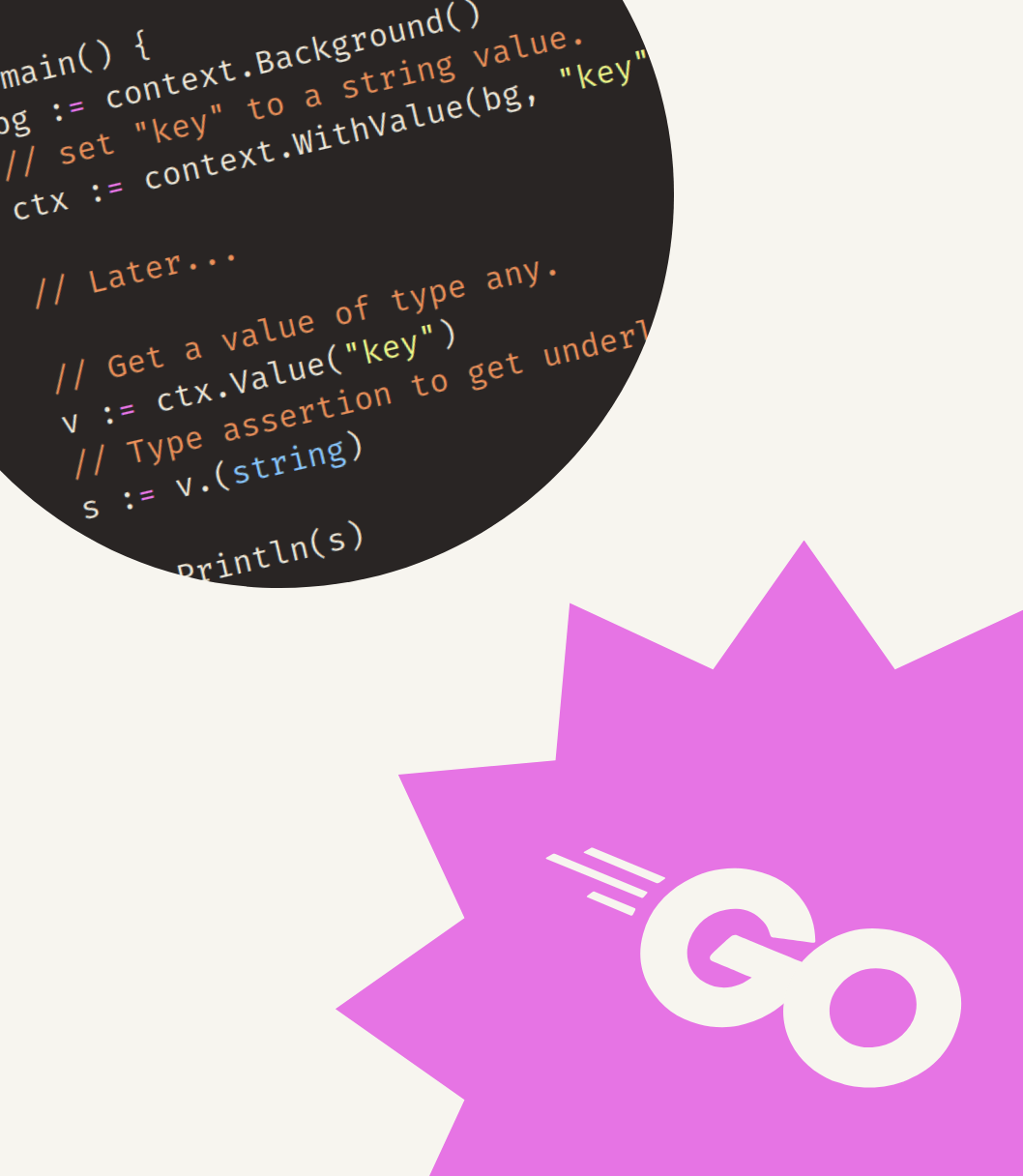
Get my free newsletter periodically*
Used by 500+ developers to boost their Go skills.
*working on a big project as of 2025, will get back to posting on the regular schedule once time allows.
