Sometimes you need to find the index of a value in a slice, and often you’re only interested in the first occurrence of that value.
This is typically done using an Index
function, which returns the index of the value in the slice or -1
when it’s not found.
Depending on your Go version you can use a different approach:
- In 1.21, you can use the
Index
function in theslices
package in the standard library. - For earlier versions, you can find the same function in the experimental
slices
package.
==
. Depending on your situation you may want to compare in a different way. The IndexFunc
function allows you to specify a custom comparison function.
If you are using a Go version that does not support generics, you can always implement your own Index
function. See the example below.
package main
import (
"fmt"
"slices"
)
func main() {
s := []string{"🌱", "🪡", "🌱"}
x := "🪡"
y := "🙈"
fmt.Println("slices.Index:")
fmt.Printf("index of \"%s\"? %v\n", x, slices.Index(s, x))
fmt.Printf("index of \"%s\"? %v\n", y, slices.Index(s, y))
fmt.Println("---")
fmt.Println("Index:")
fmt.Printf("index of \"%s\"? %v\n", x, Index(s, x))
fmt.Printf("index of \"%s\"? %v\n", y, Index(s, y))
}
// Index returns the index of the first occurrence of v in s.
// Index returns -1 if v is not found in s.
func Index(s []string, v string) int {
for i, vs := range s {
if vs == v {
return i
}
}
return -1
}
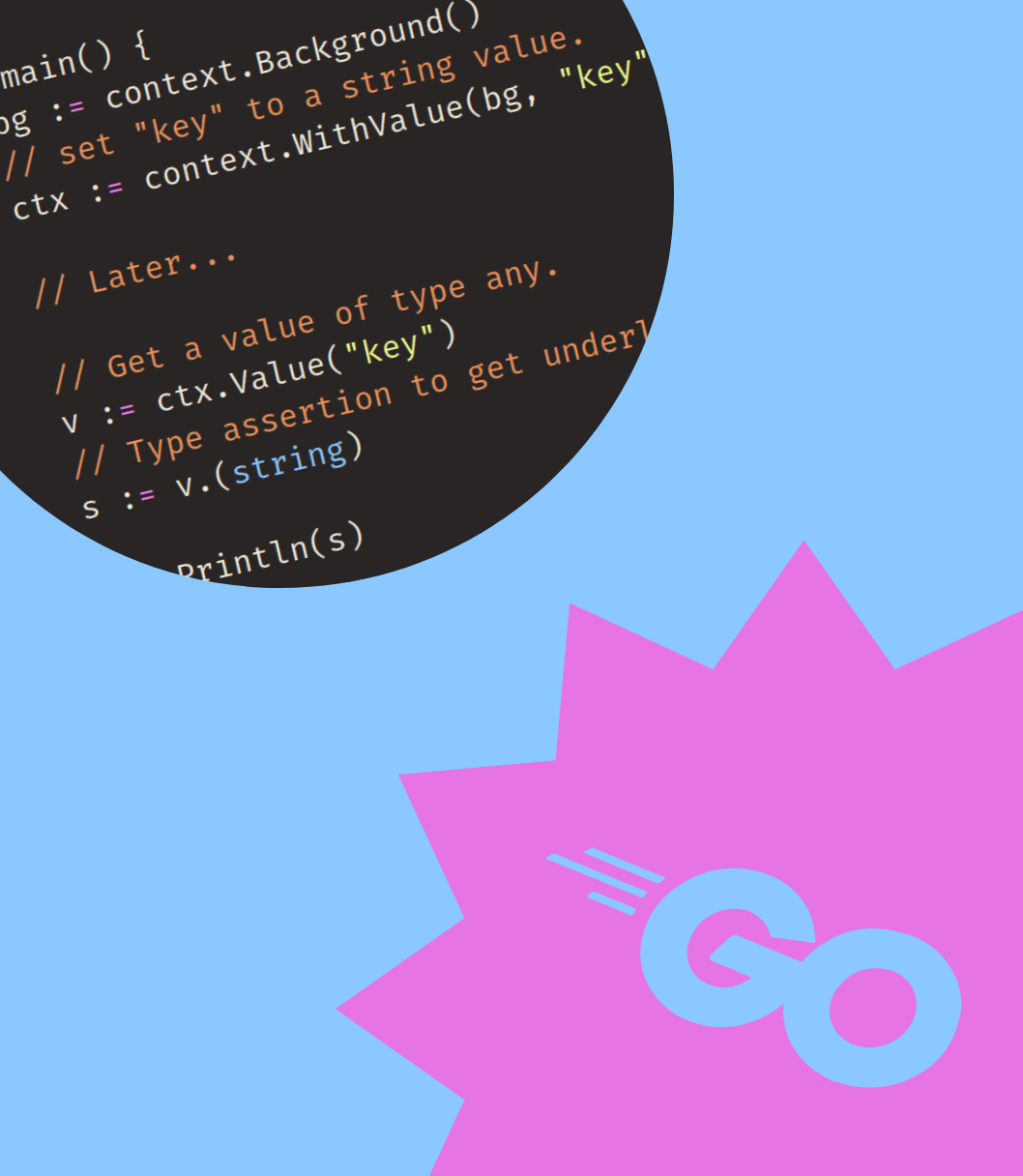
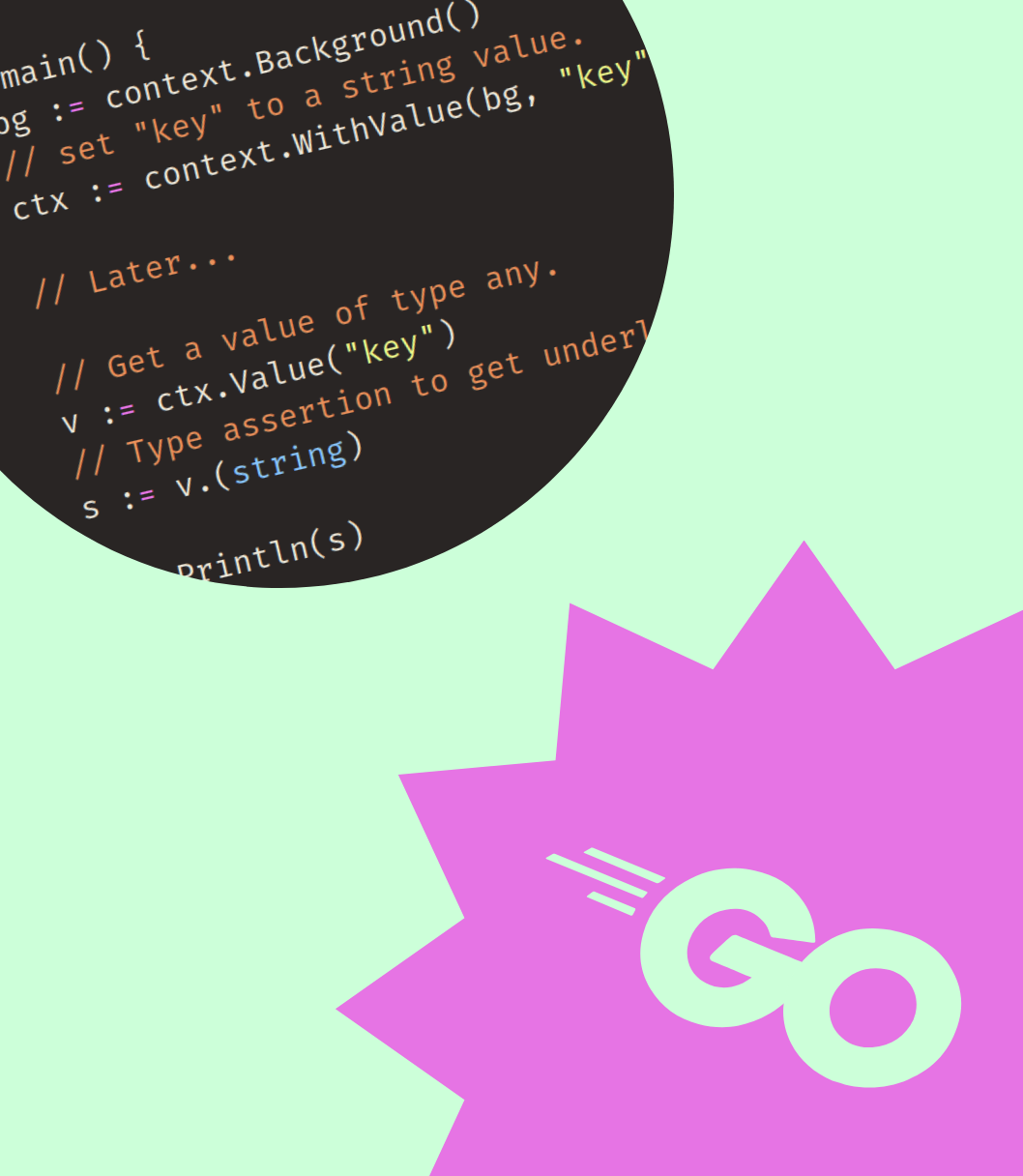
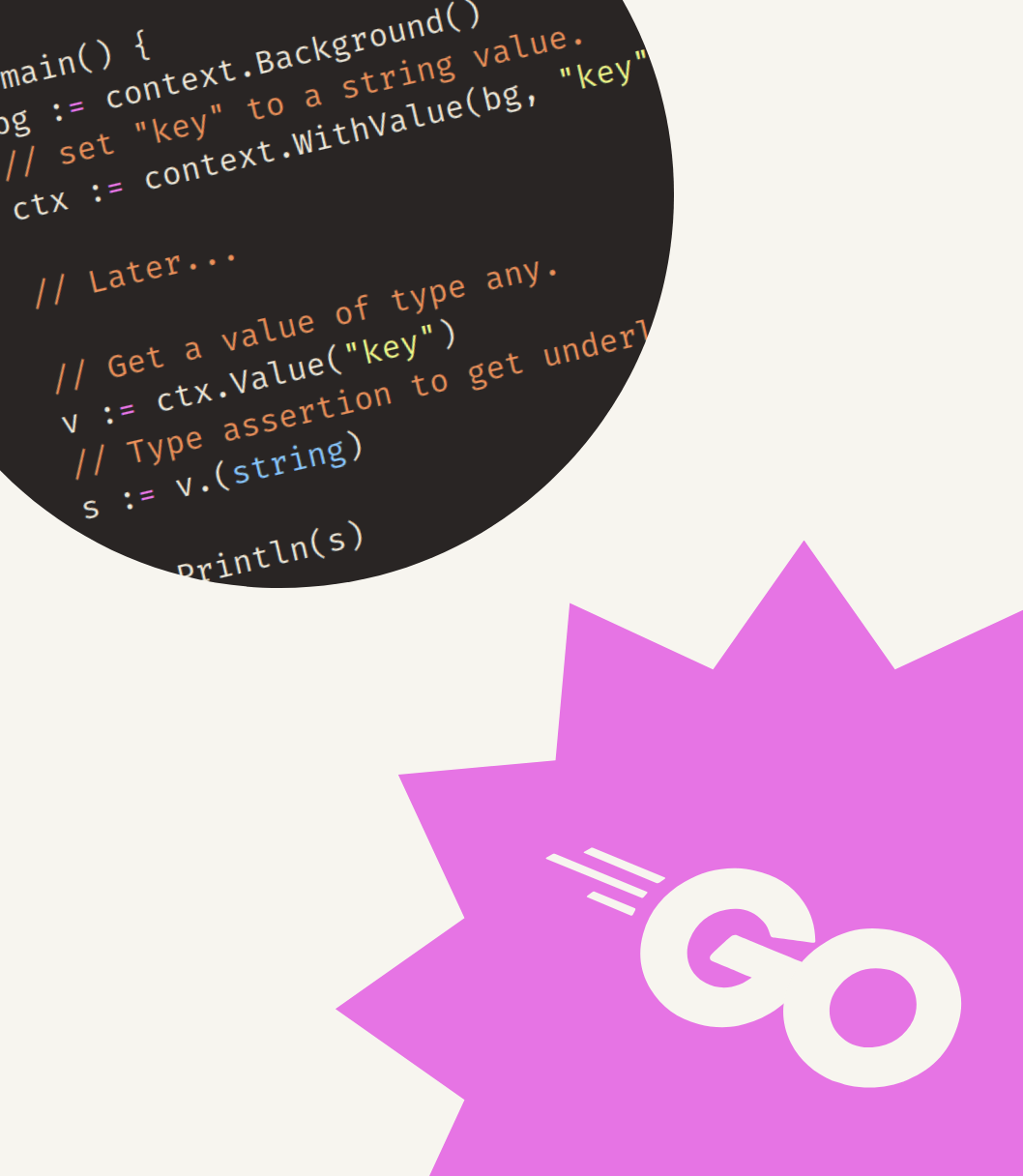
Get my free newsletter periodically*
Used by 500+ developers to boost their Go skills.
*working on a big project as of 2025, will get back to posting on the regular schedule once time allows.
